All methods
Staking
stake()
First prepares stake transaction, then signs and confirm it
Syntax:
type SetTxStageCallback = (props: {
txStage: TX_STAGE;
transactionHash?: TransactionSignature; // for TX_STAGE.AWAITING_BLOCK stage
stSolAccountAddress?: PublicKey; // for TX_STAGE.AWAITING_SIGNING stage
}) => void;
// With txStage
const { transactionHash, stSolAccountAddress } = await solidoSDK.stake({
amount: 20,
wallet: walletInstance,
setTxStage: setTxStageCallback,
});
// Without txStage
const { transactionHash, stSolAccountAddress } = await solidoSDK.stake({
amount: 20,
wallet: walletInstance,
});
// For PDA (Program Derived Address)
const { transactionHash, stSolAccountAddress } = await solidoSDK.stake({
amount: 20,
wallet: walletInstance,
allowOwnerOffCurve: true,
});
Parameters:
amount
- The amount of SOL-s which need to stakewallet
- Wallet instance from @solana/wallet-adapter-basesetTxStage?
- Optional callback for getting information about transaction stage- *
allowOwnerOffCurve?
- Allow the owner account to be a PDA (Program Derived Address), when we are creating ATA (Associated Token Account)
Return value:
type: Promise<{ transactionHash: string; stSolAccountAddress: PublicKey }>
value definition: hash of transaction got from connection.sendRawTransaction
value definition: PublicKey
class from @solana/web3.js
getStakeTransaction()
Prepares & returns stake transaction
Syntax:
const { transaction, stSolAccountAddress } = await solidoSDK.getStakeTransaction({
amount: 20,
payerAddress: new PublicKey(wallet.publicKey),
});
Parameters:
amount
- The amount of SOL-s which need to stakepayerAddress
- Address of user who is trying to make the transaction (wallet.publicKey
)- *
allowOwnerOffCurve?
- Allow the owner account to be a PDA (Program Derived Address), when we are creating ATA (Associated Token Account)
Return value:
type: Promise<{ transaction: Transaction; stSolAccountAddress: PublicKey }>
value definition: Transaction
class from @solana/web3.js
value definition: PublicKey
class from @solana/web3.js
calculateMaxStakeAmount()
Returns maximum available SOL-s to stake for given address
Syntax:
const maxStakeAmountInLamports = await solidoSDK.calculateMaxStakeAmount(new PublicKey(wallet.publicKey));
Parameters:
address
- address of user (wallet.publicKey
)
Return value:
type: Promise<number>
value definition: Value measurement is in lamports
signAndConfirmTransaction()
Signs and confirm given transaction
Syntax:
const transactionHash = await solidoSDK.signAndConfirmTransaction({
transaction: stakeTransaction,
wallet: walletInstance,
setTxStage: setTxStageCallback,
});
Parameters:
transaction
- (un)stake transaction, got fromgetStakeTransaction
wallet
- Wallet instancesetTxStage?
- Optional callback for getting information about transaction stage
Return value:
type: Promise<string>
Use transactionHash
for generating links to solana explorers. For example to https://solana.fm
UnStaking
unStake()
First prepares unStake transaction, then signs and confirm it
Syntax:
type SetTxStageCallback = (props: {
txStage: TX_STAGE;
transactionHash?: TransactionSignature; // for TX_STAGE.AWAITING_BLOCK stage
deactivatingSolAccountAddress?: PublicKey; // for TX_STAGE.AWAITING_SIGNING stage
}) => void;
// With txStage
const { transactionHash, deactivatingSolAccountAddress } = await solidoSDK.unStake({
amount: 20,
wallet: walletInstance,
setTxStage: setTxStageCallback,
});
// Without txStage
const { transactionHash, deactivatingSolAccountAddress } = await solidoSDK.unStake({
amount: 20,
wallet: walletInstance,
});
Parameters:
amount
- The amount of stSOL-s which need to unStakewallet
- Wallet instance from @solana/wallet-adapter-basesetTxStage?
- Optional callback for getting information about transaction stage
Return value:
type: Promise<{ transactionHash: string; deactivatingSolAccountAddress: PublicKey }>
value definition: hash of transaction got from connection.sendRawTransaction
value definition: PublicKey
class from @solana/web3.js
getUnStakeTransaction()
Prepares & returns unStake transaction
Syntax:
const { transaction, deactivatingSolAccountAddress } = await solidoSDK.getUnStakeTransaction({
amount: 20,
payerAddress: new PublicKey(wallet.publicKey),
});
Parameters:
amount
- The amount of stSOL which need to unStakepayerAddress
- address of user who is trying to make the transaction (wallet.publicKey
)
Return value:
type: Promise<{ transaction: Transaction, deactivatingSolAccountAddress: Publickey }>
value definition: Transaction
class from @solana/web3.js
value definition: PublicKey
class from @solana/web3.js
calculateMaxUnStakeAmount()
Returns maximum available stSOL-s to unStake for given address
Syntax:
const maxUnStakeAmountInLamports = await solidoSDK.calculateMaxUnStakeAmount(new PublicKey(wallet.publicKey));
Parameters:
address
- address of user (wallet.publicKey
)
Return value:
type: Promise<number>
value definition: Value measurement is in lamports
calculateMinUnStakeAmount()
Returns minimum available stSOL-s to unStake
Syntax:
const minUnStakeAmountInLamports = await solidoSDK.calculateMinUnStakeAmount();
Return value:
type: Promise<number>
value definition: Value measurement is in lamports
isUnStakeAvailable()
Returns unStake operation availability
Syntax:
const isUnStakeAvailable = await solidoSDK.isUnStakeAvailable();
Return value:
type: Promise<boolean>
Transaction info
getExchangeRate()
Returns exchange rate for stSOL-SOL
Syntax:
const { SOLToStSOL, stSOLToSOL } = await solidoSDK.getExchangeRate();
console.log(`1 stSOL = ~${stSOLToSOL} SOL`); // 1 stSOL = ~1.0485 SOL
console.log(`1 SOL = ~${SOLToStSOL} stSOL`); // 1 SOL = ~0.9537 stSOL
Parameters:
precision?
- the number of digits to appear after the decimal point (default = 4
)
Return value:
type: Promise<{
SOLToStSOL: number,
stSOLToSOL: number,
description: string
}>
getTransactionCost()
Returns transaction cost for given instruction
Syntax:
const { costInUsd, costInSol } = await solidoSDK.getTransactionCost(INSTRUCTION.STAKE);
console.log(`~ ${costInSol} SOL (${costInUsd})`); // ~ 0.000005 SOL ($0.00021)
Parameters:
instruction
- INSTRUCTION code
Return value:
type: Promise<{
costInUsd: number,
costInSol: number,
costInLamports: number
}>
getStakingRewardsFee()
Returns staking rewards fee percent with it description
Syntax:
const { fee } = await solidoSDK.getStakingRewardsFee();
console.log(`Staking rewards fee: (${fee})`);
Return value:
type: Promise<{
fee: string,
description: string
}>
getTransactionInfo()
Returns the united response of the previous functions (exchangeRate
, transactionCost
, stakingRewardsFee
)
Syntax:
const { exchangeRate, transactionCost, stakingRewardsFee } = await solidoSDK.getTransactionInfo(INSTRUCTION.STAKE);
console.log(`Exchange rate: ${exchangeRate.value}`);
console.log(`Transaction cost: $${transactionCost.costInUsd}`);
console.log(`Staking rewards fee: (${stakingRewardsFee.fee})`);
Parameters:
instruction
- INSTRUCTION code
Return value:
type: Promise<{
exchangeRate: {
value: number,
description: string
},
transactionCost: {
costInUsd: number,
costInSol: number,
costInLamports: number
},
stakingRewardsFee: {
fee: string,
description: string
}
}>

Lido statistics
getStakeApy()
Returns the annual percentage yield for Lido on Solana
Syntax:
import { getStakeApy as getSolidoStakeApy } from '@lidofinance/solido-sdk';
const solidoStakeApy = await getSolidoStakeApy();
console.log(`Annual percentage yield: ${solidoStakeApy}%`);
console.log(`Max APY: ${solidoStakeApy.max.apy}%`);
Return value type:
type ApyData = {
apy: number;
apr: number;
intervalPrices: {
beginEpoch: number;
endEpoch: number;
};
};
Promise<{
max: ApyData; // max apy data
lastEpoch: ApyData;
twoWeeks: ApyData;
oneMonth: ApyData;
threeMonth: ApyData;
sinceLaunch: ApyData;
}>;
Example:
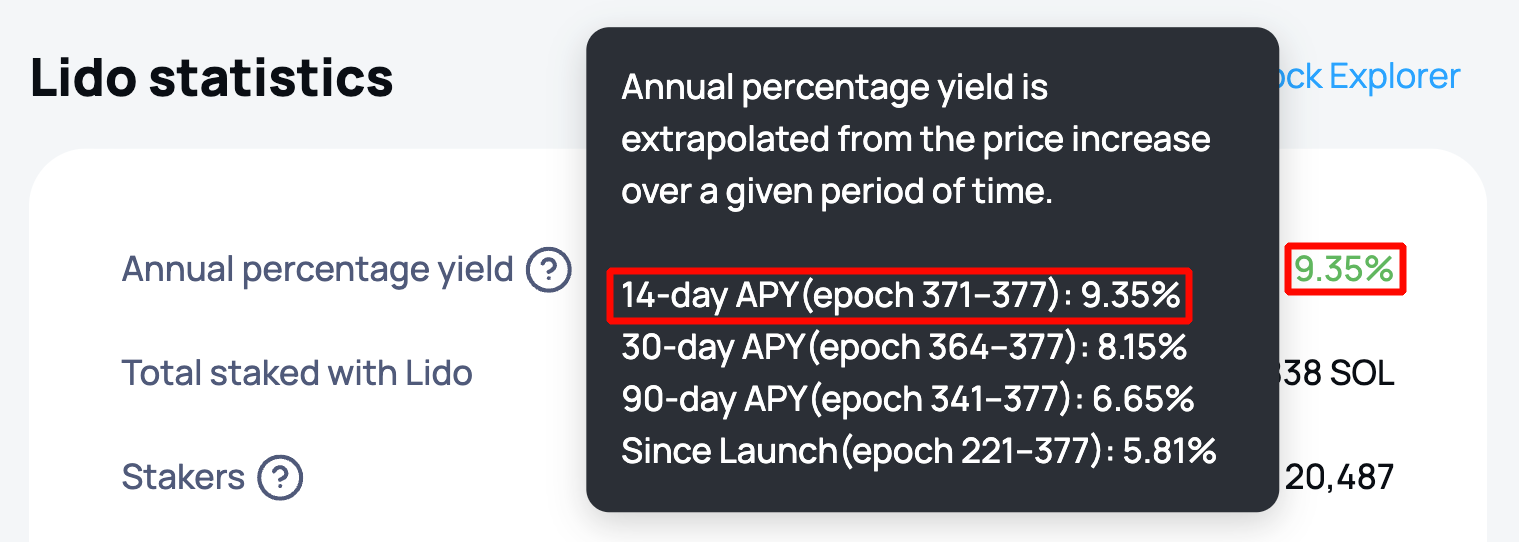
getTotalStaked()
Returns total staked SOL-s
Syntax:
const totalStaked = await solidoSDK.getTotalStaked();
console.log(`${totalStaked} SOL`); // 2620337.84 SOL
Parameters:
precision?
- the number of digits to appear after the decimal point (default = 2
)
Return value:
type: Promise<number>
getStakersCount()
Returns the number of non-empty stSOL accounts
Syntax:
const stakersCount = await solidoSDK.getStakersCount();
console.log(`Stakers: ${stakersCount.value}`); // 14965
Return value:
type: Promise<{
value: string,
description: string,
accountsTotal: number,
accountsEmpty: number,
}>
getMarketCap()
Returns stSOL market cap in $
Syntax:
const marketCap = await solidoSDK.getMarketCap();
console.log(`stSOL market cap $${marketCap}`); // $113174513
Parameters:
totalStakedInSol?
- total staked SOL-s
Return value:
type: Promise<number>
getTotalRewards()
Returns total rewards that benefited stSOL holders, in total, since we started tracking in SOL
Syntax:
const totalRewards = await solidoSDK.getTotalRewards();
console.log(`Total Rewards $${totalRewards} SOL`);
Parameters:
precision?
- the number of digits to appear after the decimal point (default = 2
)
Return value:
type: Promise<number>
getLidoStatistics()
Returns the united response of the previous functions (totalStaked
, stakersCount
, marketCap
, also apy
)
Syntax:
const { apy, totalStaked, stakers, marketCap, totalRewards } = await solidoSDK.getLidoStatistics();
console.log(`Annual percentage yield: ${apy}%`);
console.log(`Total staked with Lido: ${totalStaked.formatted}`);
console.log(`Stakers: ${stakers.formatted}`);
console.log(`stSOL market cap: $${marketCap}`);
console.log(`Total Rewards $${totalRewards} SOL`);
Return value type:
Note: here will be max APY, if you want all periods, use getStakeApy
Promise<{
apy: number,
totalStaked: {
value: number,
formatted: number,
},
stakers: {
value: string,
description: string,
accountsTotal: number,
accountsEmpty: number,
formatted: string,
},
marketCap: number,
totalRewards: {
value: number,
formatted: string,
},
}>
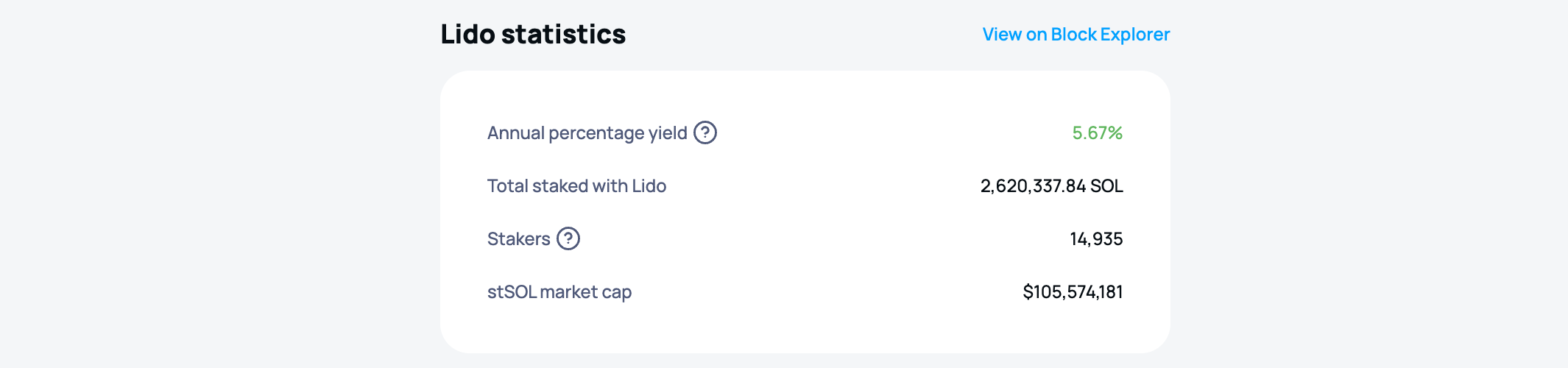
enum INSTRUCTION {
STAKE = 1,
UNSTAKE = 2,
}